I worked with the talented people at Mindnow over the course of 2023 to help them bring to life the “Mindentity”, a generative visual system representing each individual working at the company.
I was in charge of making a JavaScript library that would allow them to dynamically display Mindentities in various places of their new website and communication supports.
Concept
Mindnow already had a pretty good idea on the look of a Mindentity and the different rules driving the design when I joined the project. A Mindentity is an equidimensional grid filled with rounded squares, circles and crosses with adjustable parameters: size, radius and color.
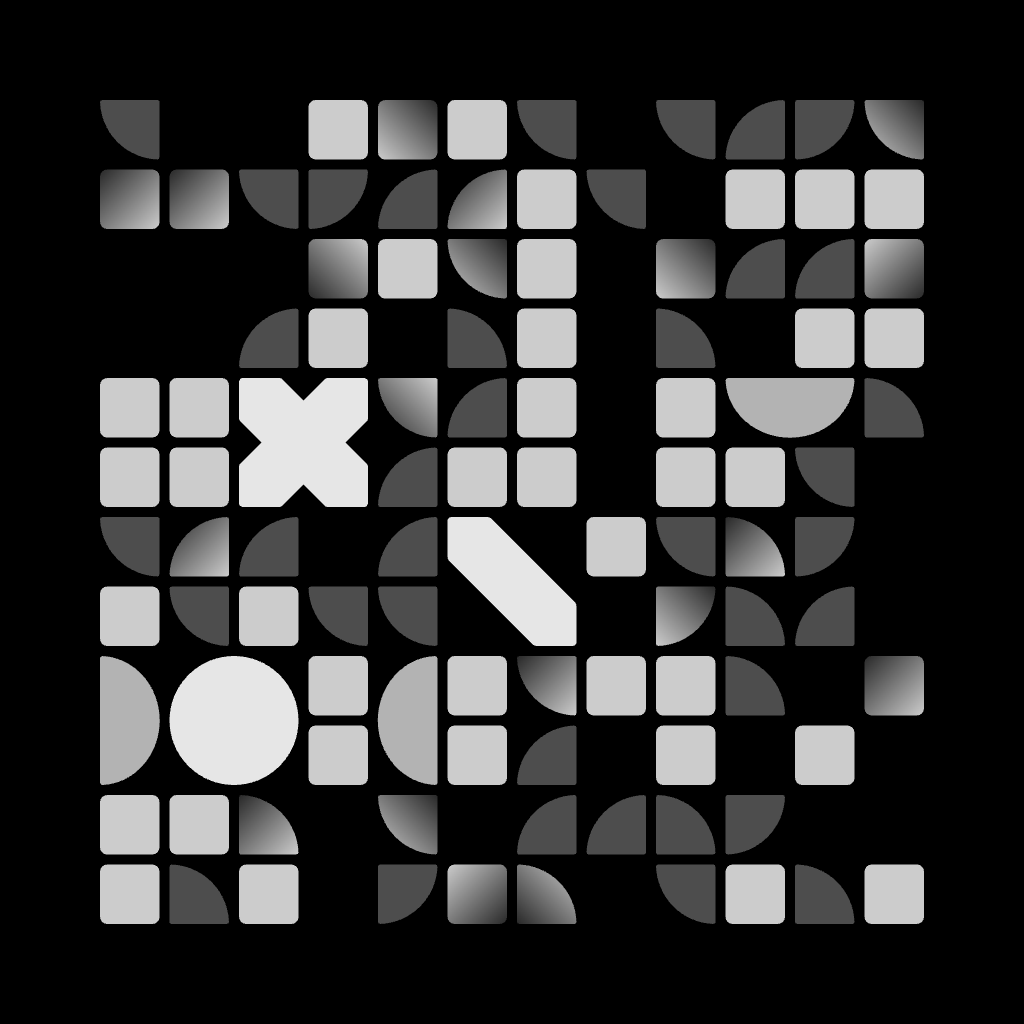
Drawing the shapes
I started working in Fragment by drawing each shape individually first. Since the Mindentities would be displayed in various sizes, I had to make sure the library could output SVG files.
I wrote a custom renderer for Fragment to display an SVG on top of the canvas I usually work with, and wrote a function that would parse and draw it on the canvas to keep the export capabilities of the tool.
I relied on the existing primitives in SVG and Canvas 2D for circles and rounded squares and wrote custom paths functions for crosses and half shapes. The most challenging aspect was the variable rounding of the shapes. Luckily, the topic was addressed in depth in these two articles:
Rounding Polygon Corners https://observablehq.com/@daformat/rounding-polygon-corners An Algorithm for Polygons with Rounded Corners https://www.gorillasun.de/blog/an-algorithm-for-polygons-with-rounded-corners/Once implemented, the shapes were all parametric and I could move onto the next step: put them on a grid.
Distributing the shapes
I began by creating a parametric grid with a variable count of cells, along with adjustable margin and gutter sizes.
Each cell of the grid keeps track of its neighbours and nodes. Once the grid is computed, the algorithm loops over cells and nodes in different steps to fill the grid following rules to avoid duplication and repetition.
Coloring the shapes
Each type of shapes has different coloring rules. Some can be filled with a linear gradient while others can only be a solid color. The distribution over the amount of gradient versus solid colors is handled through a weighted set using canvas-sketch-util .
const randomGradient = (excludes = []) => {
return random.pick(
[
'gradient-bottom-left',
'gradient-top-left',
'gradient-top-left',
'gradient-top-right',
].filter((gradient) => !excludes.includes(gradient)),
);
};
const fill = random.weightedSet([
// 80% chance of a solid color
{ value: 'black', weight: 80 },
// 20% chance of a gradient
{ value: randomGradient(), weight: 20 },
]);
The two colors could also changed, bringing more diversity into the system.
Building the Alphabet
The Mindentities are based off the first letter of an employee’s firstname. For example, Jakob Kaya would distinguish the letter J in his one.
Since we decided to keep a fixed 8x8 cells grid, I came up with a visual system directly in code that would allow me to see and adjust the 26 letters of the latin alphabet quickly.
I wrote all the letters as a grid of 8x8 emojis where 🟩 means a cell is valid and 🟥 that it should be discarded.
const A = `
🟥 🟥 🟩 🟩 🟩 🟩 🟥 🟥
🟥 🟥 🟩 🟩 🟩 🟩 🟥 🟥
🟥 🟩 🟩 🟥 🟥 🟩 🟩 🟥
🟥 🟩 🟩 🟥 🟥 🟩 🟩 🟥
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
`;
const E = `
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟥 🟥
🟩 🟩 🟩 🟩 🟩 🟥 🟥 🟥
🟩 🟩 🟩 🟩 🟩 🟥 🟥 🟥
🟩 🟩 🟥 🟥 🟥 🟥 🟥 🟥
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
`;
const H = `
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟩 🟩 🟩 🟩 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
🟩 🟩 🟥 🟥 🟥 🟥 🟩 🟩
`;
Once this was done, I was able to generate the entire alphabet and improve the readability of each letter by adjusting cells in the code.
Avoiding repetitions
Since people at Mindnow could share the same letter, I used the full name of an individual as the seed of the Pseudo Random Number Generator to create truly unique Mindentities.
Conclusion
The final step was to turn this into an API so Mindnow developers could integrate it easily on their website.
I had a blast bringing this visual system to life for Mindnow and I hope you will like this project as much as I do! I was also very pleased to use Fragment for the entire process of this project, which made the development experience a breeze.
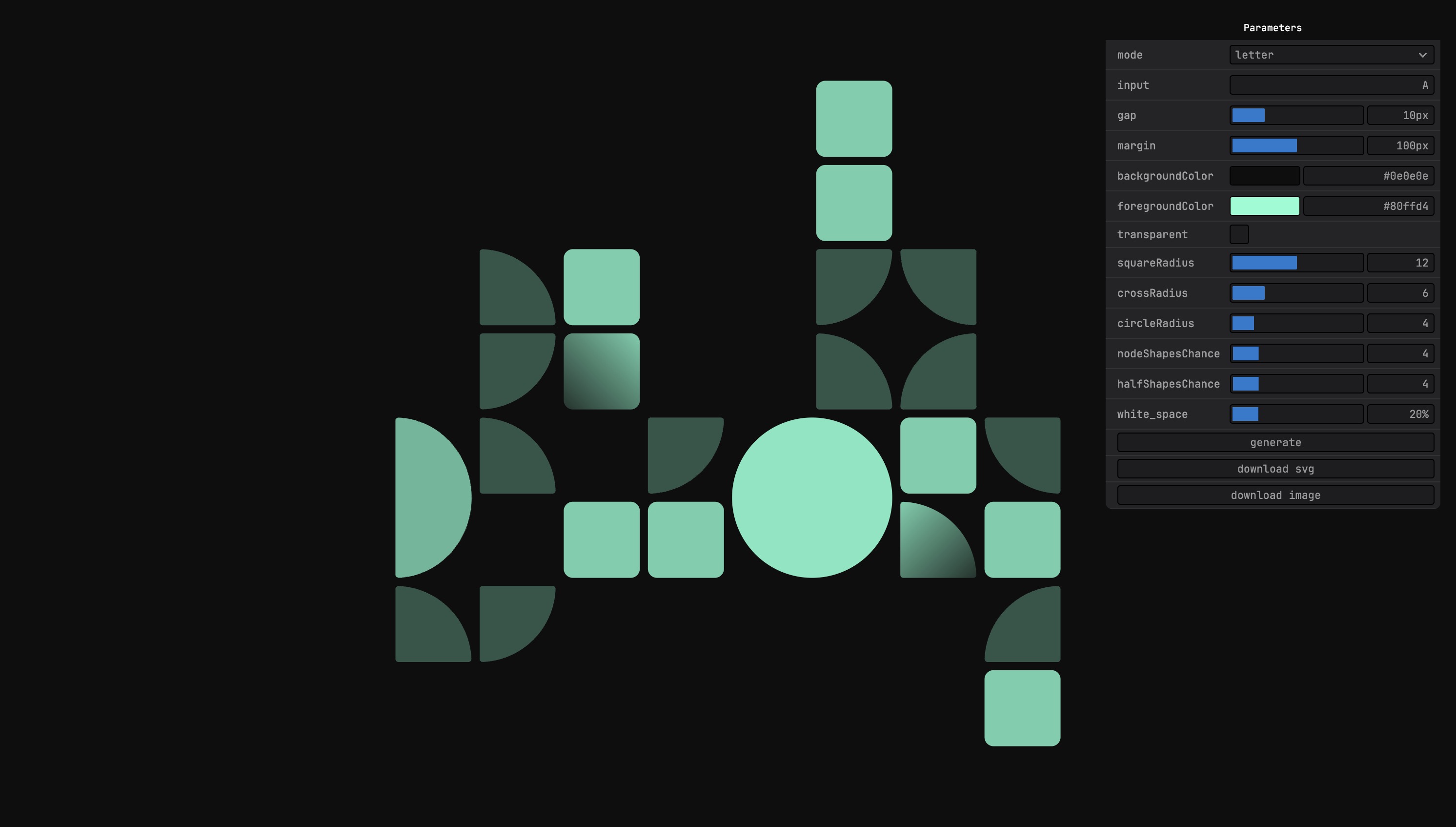
You can see the project live on mindnow.io .
Read more
- Institute of Design : three experiments around its visual language
- Fragment : an open-source web development environment for creative coding.